Python: Draw SVG curves in PyGame, Tkinter, and Turtle Graphics
Drawing SVG paths in Python, for PyGame, Tkinter, & Turtle Graphics
Python has rich GUI and graphics options. Some of them are built-in such as Tkinter and Turtle Graphics. Others are from package modules like PyGame, PyQt, Matplotlib.
Webpages began with primitive graphics ability such as animated gifs, images, and java applets, but now has dynamic JavaScript, CSS, and especially Scaled Vector Graphics (SVG).
SVG Paths are great for defining more complex drawings that combine lines, ellipses, cubic and quadratic Bezier curves. SVG is also a vector drawing system rather than a pixel system. That means that you can scale them from small to large, and they are always smooth.
Below are several code samples to draw a SVG continuous curve using a simple Python module: svg.path
. (ref the github page for svg.path). This package does not implement all of the SVG capabililites. But it is useful for simple things.
This code shows you how to use this module to draw in PyGame, TKinter, and Turtle Graphics. From these examples, you should be able to adapt it to many other GUI or drawing libraries.
Install the svg.path module in a terminal with the command python3 -m pip install svg.path
(insead of python3
, your system may have just python
in the command).
svg.path draw paths with lines, cubic and quadratic bezier curves, and ellipses. It will create a path by a series of method calls, or by using a path string as specified for the SVG d attribute for CSS: see Mozilla SVG curves tutorial
Quadratic Paths:
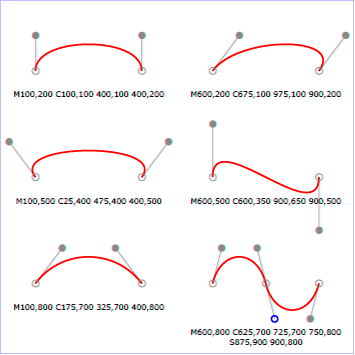
Cubic Path:
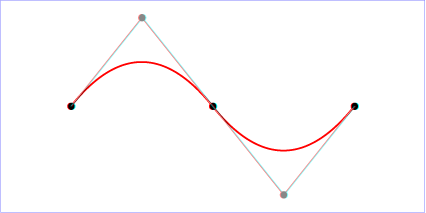
Ellipse (Arc) Path:
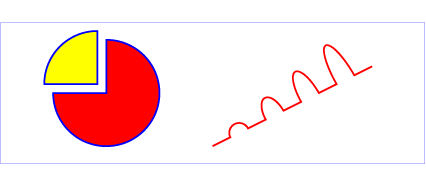
To use the svg.path package for drawing d attribute path strings:
from svg.path import Path, Line, Arc, CubicBezier, QuadraticBezier, parse_path
d = "M100,200 C100,100 250,100 250,200 S400,300 400,200"
x = parse_path(d)
The code above would define a path object named x
that would represent the path defined by the string in d
. This path defines a move to 100,200 and then two cubic curves:
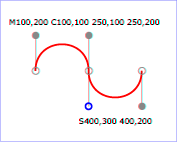
Now that we have a path object: x
We can call the point
method to get a x,y
position on the path expressed as a Python complex number for a percent distance along the path expressed as a fraction from 0.0 to 1.0.
p = x.point(0.5) # half way
would return the point half way along path: the (250+200j)
(find out about python complex numbers)
Note that the makers of the svg.path module decided to use complex number type to store the x and y values. It is simple to use for storing two real numbers as a pair, most python programmers would choose a two-value tuple or a named tuple for this.
p2 = (p.real, p.imag) # this converts the complex number p to a tuple (x, y) value p2
video fo getting SVG from Inkscape to use in code for PyGame code
Code for PyGame
Code for Tkinter
Code for Turtle Graphics
HAVE FUN!
– Professor Gerry Jenkins