PyGame Blit Method Explained
Contents
Understanding the basic PyGame blit method parameters and use via 4 code samples with an associated youtube video
- Two argument blit from image to screen (surface1 to surface2 at location)
- Three argument blit from area of surface1 to location of surface2
- Copy from area of surface to another area of same surface
- Using the special_flags parameter to do blending
Blit Background
Bit blitting dates to the first window-based GUI on the Xerox Alto computer in 1973. In the 80’s, Bit Blitters were built into video game hardware. For games, it is the primary way sprite images were efficiently drawn into the display. Soon after blitter code became part of the operating system software of both Apple and Microsoft first graphic user interface operating systems.
In PyGame, blit is the workhorse method to copy from surface to surface. Anything you draw or any visual resource you use gets rendered onto a pixel rectangular surface object. The main working buffer associated with the display is also a surface.
The blit method copies an area of one surface to an area of another surface with an option of merging the source and destination pixels with certain modes to ‘blend’ the colors or brighten or darken them.
It is important to understand that blit always copies pixels from one surface to another. It does shrink or expand any pixels that are copied.
Also, any pixels that are outside the destination surface or any clipping region are not copied.
The syntax for Surface blit method is listed under the PyGame Surface Documentation as follows:
surface_object.blit(source, dest, area=None, special_flags=0) -> Rect
The return value is a Rect in the coordinate system of the destination surface object. It gives you the rectangle of effective pixels modified in the destination surface. The area designated in the call to blit will be clipped to the boundary of the destination surface or its clipping area.
The code for this article is located at github.com/gerryjenkinslb/blit_article
Basic blit: just the required parameters: source and dest
asurface.blit(source, dest)
This first picture demonstrates a pixel blowup to show the action of a simple blit from a image to the screen, and how the source pixels are copied pixel by pixel.
In the picture below.
screen is the display surface, source is img, which is a surface loaded from an image file, and dest is the tuple: (10,10), the x, y position of the upper left corner of the copied source surface.
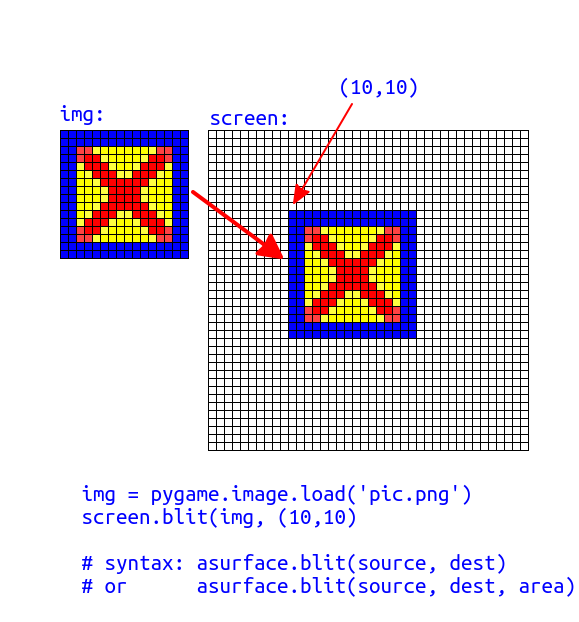
Note that the dest parameter can also be a PyGame Rect, and the location will be the upper left corner of this Rect, the width and height of the Rect are ignored.
This next picture is the result of the program three_blits.py. When you run this code, the first output will be this simple two parameter blit:
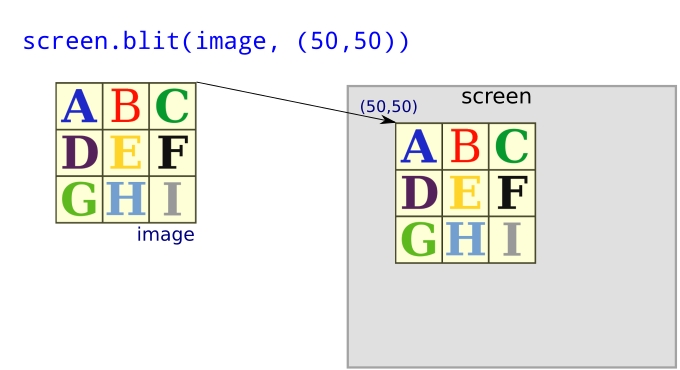
Bilt with area parameter
asurface.blit(source, dest, area)
The area is a Rect that represents a smaller portion of the source Surface to draw to the target surface. It is in the coordinate system of the source surface
Here is an image that shows copying a smaller area of from the source image.
*The new area is copied below the original when you hit a key on the three_blits.py program.
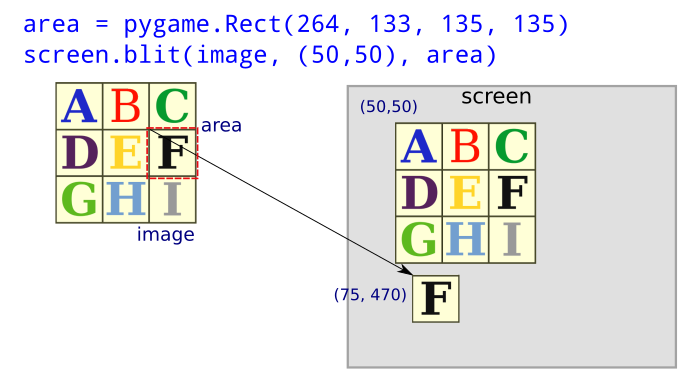
Bilt with area parameter but from the screen surface to itself
This last example is shown in the three_blits.py program when you hit a key again. It first draws a yellow rectangle around the area we are going to copy. Then it uses blit with a area parameter to copy from the screen where we drew the yellow rectangle to are are below and to right or the two previous examples.
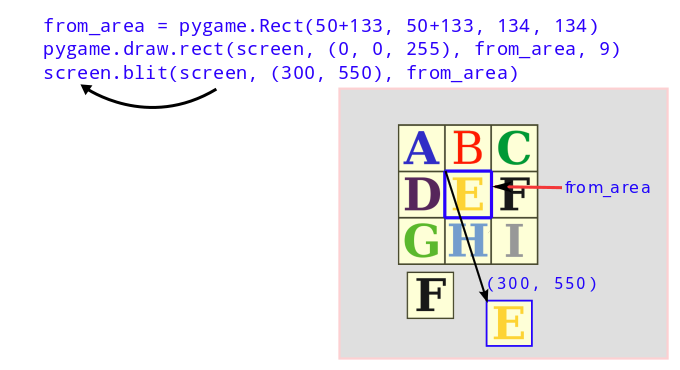
The optional special_flags parameter
asurface.blit(sourc, dest, special_flags=pygame.BLEND_ADD) # example with blend add option
The special_flags optional parameter can be used for blending two images in different ‘modes’ and for other advanced options.
Most of these options are beyond the scope of this article.
The simplest of these flags are
PyGame.BLEND_ADD:
Add each color channel (RGB) value of the source to the destination. Lightens the image.
PyGame.BLEND_SUB:
Subtracts each color channel (RGB) value of the source from the destination. Darkens the image.
Example Blend
Here is a sample of using the ADD blend with a mask that increases the primary colors red, green, and blue by hex 80 which is 50% of each full color in different areas. The the last area adds hex 80 to all three colors.
Note that I have annotated the hex values of each mask. You can see that the mask brightens each area where applied. For the three primary color masks, it just increase the red, green, and blue component, intensifying each color. The last mask brightens all colors.
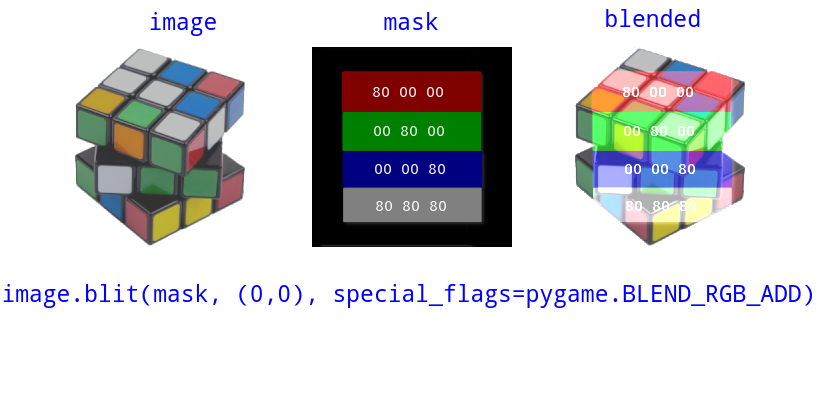
References:
PyGame Surface Blit Documetation
gimp.org Layer Modes to combine images: Overview of blending modes with good explanation of Additive, Subtract, and Multiply.
Lighting in PyGame (Tutorial): Use in a Game for Particles see youtube.com
sourcegraph search for code bases that use the pygame special_flags parameter.
CODE FOR ARTICLE at github.com/gerryjenkinslb/blit_article